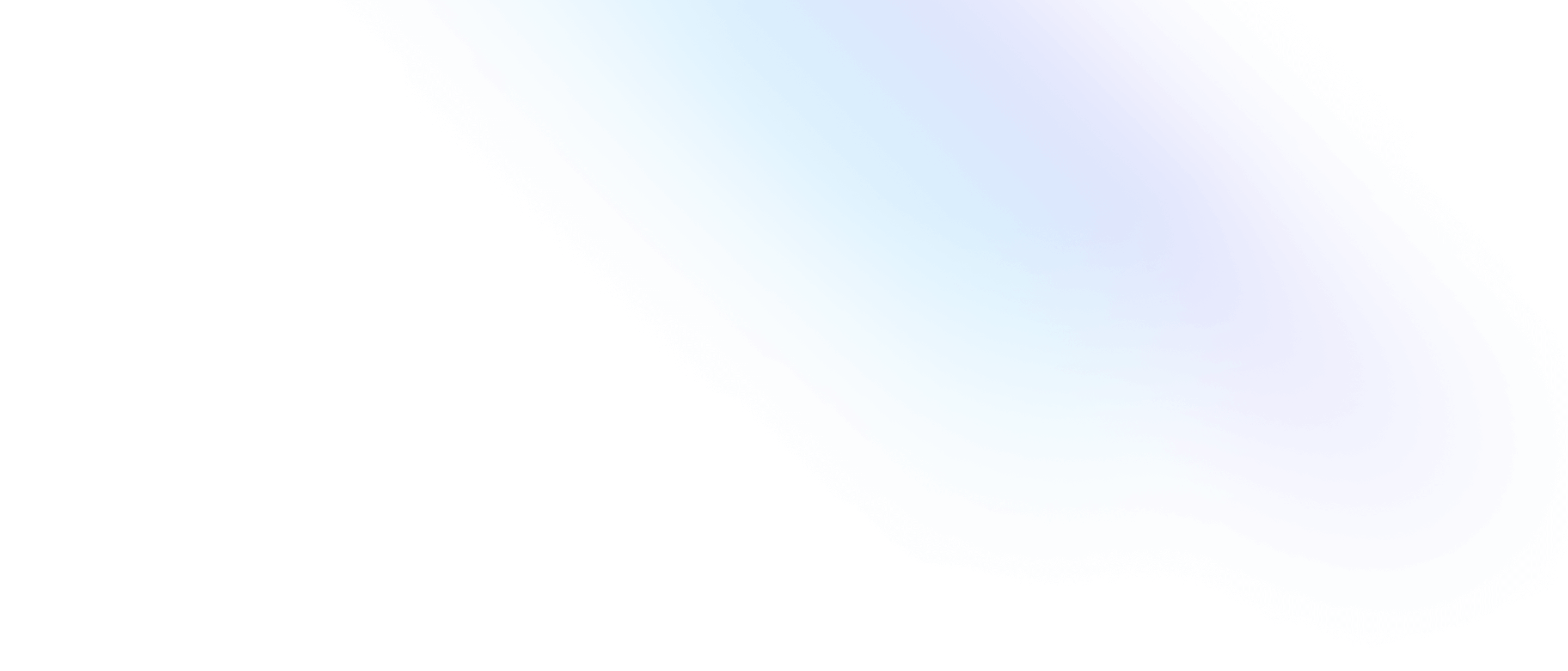
Introduction
Get started with the Luna API
Our API enables you to programmatically access people and company data through our JSON based API.
Getting Started
The Luna API is organized around REST. Our API has predictable resource-oriented URLs, accepts form-encoded request bodies, returns JSON-encoded responses, and uses standard HTTP response codes. You can use the Luna API with an API key generated via your account's API page. Any member of the team can leverage the API using any of the account's API keys.
These docs are a reference for the latest version of the API.
Authentication
Authorizing Requests
The Luna API uses API keys to authenticate requests. You can view and manage your API keys in your Luna API keys page. Your API keys carry many privileges, so be sure to keep them secure! Do not share your API keys in publicly accessible areas such as GitHub or client side code.
All API endpoints require a valid API key. The best and most secure way to pass your API key along is to do so as a bearer token through the standard authorization header.
$ curl -H "Authorization: Bearer YOUR-KEY" ...
Please note, if you are using JavaScript you may need to set the credentials request option to "include" to ensure the authorization header is sent.
If your API key is missing or invalid the API request will return an error. The error code returned will
be a 401
.
Responses
Response Formats
All error responses have the following format.
{
"meta": {
"id": "foobar"
},
"params": {
"linkedin_username": "wozniaksteve"
},
"status": 404,
"error": {
"type": "not_found",
"message": "There were no records found matching your request."
}
}
The meta
field is any meta data that was sent with the
request. Likewise, the params
field are the original
parameters from the request.
The error type can be one of the following:
-
invalid_request_error
expected parameter was missing or invalid. -
not_found
enrichment didn't match a record or search returned no results. -
api_error
internal server error, something happened on our end. authentication_error
missing or invalid API key.-
payment_required
plan lapsed or credits exhausted.
Successful responses have similar format.
{
"meta": {
"id": "foobar"
},
"params": {
"linkedin_username": "billgates"
},
"status": 200,
"data": { ... }, /* single object (enrichment) or array of objects (search) */
"total": integer /* search endpoints only */
}
Response Codes
Our API leverages standard HTTP response codes for every request. In addition to using HTTP codes we also return a JSON-based response (formats can be found above).
Here's a breakdown of error codes you may see and what they mean:
200
successful response.-
400
invalid request, one or more parameters missing. 401
unauthorized, invalid or missing api key.-
402
payment required, plan lapsed or credit limit reached. -
404
not found, enrichment didn't match a record or request requirements failed. 405
method not allowed.500
internal server error.
Credits
Credit Information
All responses return credit information. This information lets you know how many credits the request used and how many credits are remaining.
Credit information is returned as response headers:
-
X-Credits-Spent
how many credits the request consumed. -
X-Credits-Remaining
how many credits are remaining on your account. -
X-Credits-Limit
the total credit limit for the account.
Credit Usage
Our API uses a fractional credit system.
-
People Enrichment
each successful person enriched consumes 1.0 credit. -
People Search
for each person in the result 1.0 credit is consumed. -
Company Enrichment
each company enriched consumes 0.25 credit. -
Company Search
for each company returned 0.25 credit us consumed.
People — Enrich
Enrich People
Our person enrichment API enables you to easily look up a person's contact information.
Enrichment
The person enrichment endpoint takes a LinkedIn username, LinkedIn URL, or an email address and returns a person's profile information with contact information. Contact information is defined as a personal email, work email, or a phone number.
The endpoint expects at least one of the following query parameters:
linkedin_username
a public LinkedIn identifier.linkedin_id
a numeric LinkedIn identifier.linkedin_url
a complete LinkedIn profile URL.-
email
any email address associated with the person. uuid
Luna's UUID for a given person.
More than one parameter may be given. The first parameter that matches a person will be used. A single credit will be consumed if the query matches a person. If no person is matched a 404 response will be returned.
Optionally, you can specify a set of requirements using the
require
parameter. You can specify a comma separated list
of required response fields. Any of these fields can be specified:
work_email
personal_email
phone_number
If the requirements are not met the response will be a 404 (not found).
GET https://www.lunadata.io/api/v1/person/enrich?linkedin_username=wozniaksteve
{
"status": 200,
"data": {
"uuid": "a2528c93-c11c-4d30-b9c2-1d413ec93bb3",
"first_name": "steve",
"last_name": "wozniak",
"location": "palo alto, california",
"country": "united states",
"title": "founder",
"company": "apple",
"industry": "technology",
"personal_email": "*****@icloud.com",
"work_email": "*****@apple.com",
"phone_number": null,
"linkedin_username": "wozniaksteve"
}
}
A bulk enrichment can be performed as well. When performing a bulk request the request's content type
should be application/json
and the parameters should be
specified in the request body using JSON.
POST https://www.lunadata.io/api/v1/person/enrich/bulk
With the following request body:
{
"requests": [
{
"params": {
"linkedin_url": "linkedin.com/in/wessels-smith-bab84996"
},
"meta": {
"my-id": "some-internal-id"
}
},
{
"params": {
"email": "wozniak.steve@icloud.com"
}
}
]
}
The response follows a similar format to the single enrichment response.
{
"status": 200,
"data": [
{
"status": 404,
"meta": {
"my-id": "some-internal-id"
},
"params": {
"url": "linkedin.com/in/wessels-smith-bab84996"
},
"error": {
"type": "not_found",
"message": "There were no records found matching your request."
}
},
{
"status": 200,
"meta": null,
"params": {
"email": "wozniak.steve@icloud.com"
},
"data": {
"uuid": "2daa700e-ab74-4be9-bf88-dd1b8a94efcd",
"first_name": "steve",
"last_name": "wozniak",
"location": "palo alto, california",
"country": "united states",
"title": "co-founder",
"company": "apple",
"industry": "technology",
"personal_email": "*****@icloud.com",
"work_email": "*****@apple.com",
"phone_number": null,
"linkedin_username": "wozniaksteve"
}
}
]
}
People — Search
Discover People
Search finds one or more person matching your search parameters.
Searching
The person search endpoint takes one or more search criteria and returns a set of people that match. All matches are returned as complete people with contact information. Contact information is defined as a personal email, work email, or a phone number.
The endpoint expects one or more of the following search parameters:
first_name
the person's given name.last_name
the person's family name.location
a location (city, state).country
a country (ex: united states).industry
a given industry.title
the person's current job title.company
the person's current company.
Each parameter is considered together. For example, if title is "software engineer" and location is "palo alto" then the query looks for people with the title AND location that match. If duplicate parameters are provided they will be considered as an "OR". For example, if title is "software engineer" and a second title is "software developer" then it will look for people that match "software developer" OR "software engineer".
If no people match the search criteria then a 404 response is returned.
There are a few optional parameters that can be used to control responses:
limit
optional, default is 10, can be 1 to 25.-
offset
optional, default is 0, can be from 0 to 9999.
GET https://www.lunadata.io/api/v1/person/search?first_name=steve&last_name=wozniak&limit=1
{
"status": 200,
"meta": {
"first_name": "steve",
"last_name": "wozniak",
"limit": "1",
"offset": "0"
},
"data": [
{
"uuid": "2daa700e-ab74-4be9-bf88-dd1b8a94efcd",
"first_name": "steve",
"last_name": "wozniak",
"location": "palo alto, california",
"country": "united states",
"title": "co-founder",
"company": "apple",
"industry": "technology",
"personal_email": "*****@icloud.com",
"work_email": "*****@apple.com",
"phone_number": null,
"linkedin_username": "wozniaksteve"
}
],
"total": 1
}
Note, a single credit will be consumed for each person that is returned. If no people are found a 404 response will be returned.
Company — Enrich
Enrich Companies
Our company enrichment API enables you to easily fill in missing company information.
Enrichment
The company enrichment endpoints take enrichment parameters such as a company's name or website URL and returns the matching company profile.
The endpoint expects one of the following query parameters:
name
the company's name.url
the company's official website URL.linkedin_url
the company's LinkedIn URL.-
linkedin_id
the numeric ID for the company's LinkedIn profile. uuid
Luna's UUID for a given company.
More than one parameter may be given. The first parameter that matches a company will be used. If no company is matched a 404 response will be returned and no credit is consumed. If a company is returned 0.25 credit will be consumed.
GET https://www.lunadata.io/api/v1/company/enrich?linkedin_id=19213208
{
"status": 200,
"data": {
"uuid": "fe6ad720-8c76-45c5-a794-1c92cfea6270",
"name": "nymeria",
"url": "nymeria.io",
"size": "1-10",
"founded": "2017",
"industry": "computer software",
"location": "cedar city, utah",
"country": "united states",
"linkedin_id": "19213208",
"linkedin_url": "linkedin.com/company/nymeria"
}
}
A bulk enrichment can be performed as well. When performing a bulk request the request's content type
should be application/json
and the parameters should be
specified in the request body using JSON.
POST https://www.lunadata.io/api/v1/company/enrich/bulk
With the following request body:
{
"requests": [
{
"params": {
"linkedin_id": "19213208"
},
"meta": {
"my-id": "123"
}
},
{
"params": {
"name": "microsoft"
}
},
]
}
The response follows a similar format to the single enrichment response.
{
"status": 200,
"data": [
{
"status": 200,
"meta": {
"my-id": "123"
},
"params": {
"linkedin_id": "19213208"
},
"data": {
"uuid": "fe6ad720-8c76-45c5-a794-1c92cfea6270",
"name": "nymeria",
"url": "nymeria.io",
"size": "1-10",
"founded": "2017",
"industry": "computer software",
"location": "cedar city, utah",
"country": "united states",
"linkedin_id": "19213208",
"linkedin_url": "linkedin.com/company/nymeria"
}
},
{
"status": 200,
"meta": null,
"params": {
"name": "microsoft"
},
"data": {
"uuid": "a82b358b-5706-4289-85e5-354f45af82be",
"name": "microsoft",
"url": "microsoft.com",
"size": "10001+",
"founded": "1975",
"industry": "computer software",
"location": "redmond, washington",
"country": "united states",
"linkedin_id": "1035",
"linkedin_url": "linkedin.com/company/microsoft"
}
}
]
}
Company — Search
Discover Companies
Search finds one or more companies matching your search parameters.
Searching
The company search endpoint takes one or more search parameters and returns a set of companies that match. All matches are returned as complete company profiles.
The endpoint expects one or more of the following search parameters:
name
a company name.location
a location (city, state).country
a country (ex: united states).industry
an associated industry.size
a given employee size.
Each parameter is considered together. For example, if name is "microsoft" and location is "washington" then the query looks for companies with the name AND location that match. If duplicate parameters are provided they will be considered as an "OR". For example, if name is "microsoft" and a second name is "microsoft, inc" then it will look for companies that match either name.
If no companies match the search criteria then a 404 response is returned.
There are a few optional parameters that can be used to control responses:
limit
optional, default is 10, can be 1 to 25.-
offset
optional, default is 0, can be from 0 to 9999.
GET https://www.lunadata.io/api/v1/company/search?name=nymeria&limit=1
{
"status": 200,
"meta": {
"name": "nymeria",
"limit": "1",
"offset": "0"
},
"data": [
{
"uuid": "fe6ad720-8c76-45c5-a794-1c92cfea6270",
"name": "nymeria",
"url": "nymeria.io",
"size": "1-10",
"founded": "2017",
"industry": "computer software",
"location": "cedar city, utah",
"country": "united states",
"linkedin_id": "19213208",
"linkedin_url": "linkedin.com/company/nymeria"
}
],
"total": 1
}
Note, 0.25 credit will be consumed for each company that is returned. If no companies are found a 404 response will be returned and no credit will be consumed.